Exception Handling and Logging basics
- DigitalLiv
- Jan 14, 2022
- 3 min read
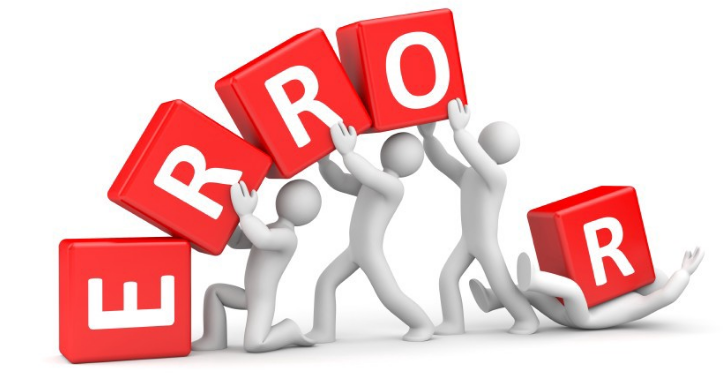
Hey kids!! Let's go over some basics for exception (error) handling and logging. This is legit the hill I will die on when I'm debating if code is good or not. If the code does not have error handling and GOOD logging, it's not ready for production.
When you add error handling and logging to your code, you make it a million times easier to pinpoint the actual error instead of having to dig around and guess. So, how do we know if our code errors?
We add Try Catch Finally blocks, with the error logging inside the Catch section. Depending on your preferred method of notification, you may insert a log row to a database, or send an email, or push a message to an API endpoint that routes the error to the appropriate notification type. Any number of ways are available to notify yourself or your team of an error. It will hopefully help reduce these for you and your team ....
It is prudent to discuss there are different logging levels you should use. These are my own personal levels I use, but you can use whatever works best for you and your organization.
Fatal
This is a 'something is down or failing' error
This should always be logged with the most amount of detail as possible
You should 100% have a notification set, as it is an emergency
Error
This is your 'errors that should not happen', such as a calculation function failed to give the cost of your product plus tax so the user sees an error instead of their total cost
This should always be logged with the necessary detail for the surrounding code
Generally, this should also have notification, but set as a high level notice
Warning
This is a notice that something happened you weren't expecting, but it won't error your system, such as a user tries to login but the username password doesn't match
This type doesn't need notifications, but maybe there is monitoring set to send these errors to a dashboard for review daily, or even weekly
Informational
This type of log may be used for extra testing or logging user actions
No notices or monitoring should be used for these. If a developer needs the information, they can go into the logging system and retrieve the needed data
When you log errors, you are doing everyone a favor around you, including yourself. Anyone who reports an error or any developer trying to find an error in your code while you're on vacation will appreciate the ease of reading your detailed error logs. It could save you a frantic call from a fellow dev when you're just trying to get a drink in your hand and toes in the sand.
When something goes wrong in your code (yes I said WHEN), your superior logging skills will shine! It can save you hours of troubleshooting and down time with your manager breathing down your back.
Add a Try Catch block around every method and/or function, especially the ones that could fail, like math calculations or missing null checks. Add a logging message, similar to the example below. It has the variable used in the method, the method name it is in, and the system message. It is also normal to add an error number rather than the method name so you can search your code with the unique number and quickly find the error. This is helpful in case a method name changes (shouldn't happen, but it does).
catch (Exception ex)
{
LoggerService.LogToSystem("The variable a = " + a + ". The method to review is MethodName. The system error captured is " + ex.Message)"
return false;
}
Obviously, the user does not need to see this full error, so you log this error and show the user a human readable error based on whatever is happening. Usually you would disply the ex.Message to the user or something you craft that makes sense to the user.
Here is an example of a full Try Catch Finally with an example log in it.
try
{
CODE
return true;
}
catch (Exception ex)
{
LoggerService.LogToSystem("The variable a = " + a + ". The error code is MGF3423. The system error captured is " + ex.Message, ex, request, Logging.LogLevel.Error, HttpContext.Current.User);
return false;
}
finally
{
//do clean up
request.close():
}
There are tons of ways to log your errors, just make sure they are easy for you to decipher when something goes wrong. If your logging is robust enough, you can quickly determine if it's a true issue or just an informational item that was set as an error. It is also good practice to combine your logging with feature flags so you can immediately turn off code that is erroring while you investigate and redeploy.
Cheers!
Comments